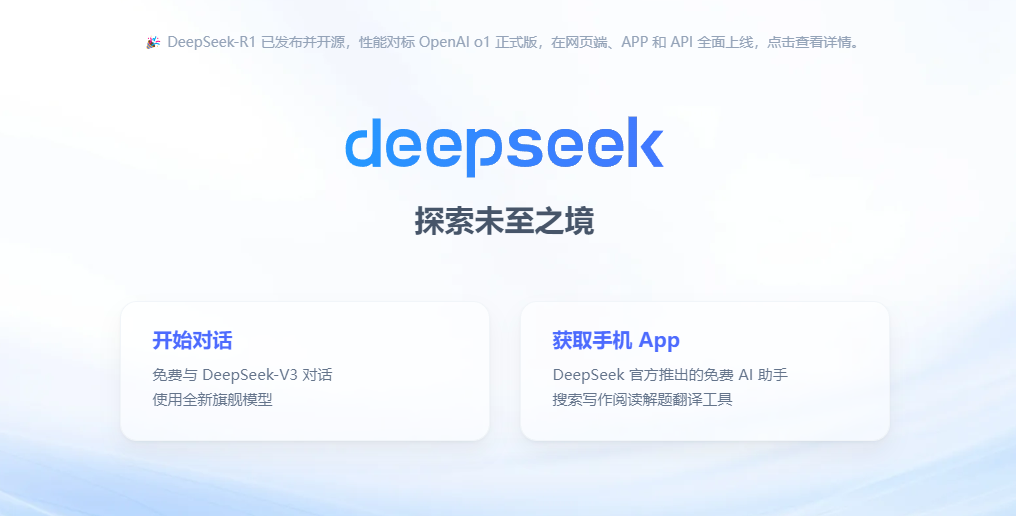
DeepSeek目前非常流行,有些网站主想通过在自有网站集成DeepSeek的方式来引流,接下来给大家提供一个前后端分离的示例,前端负责交互,后端负责调用DeepSeek的API。
- 注册DeepSeek开发者账号
- 申请API访问权限
- 在测试环境完成集成
- 申请商业部署授权
- 部署负载均衡和监控系统
第一步 官方服务接入
DeepSeek官方提供API调用服务,建议通过以下方式接入:
// 前端调用示例(需配置反向代理保护密钥)
async function queryDeepSeek(prompt) {
const response = await fetch('/api/deepseek-proxy', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${process.env.DEEPSEEK_API_KEY}`
},
body: JSON.stringify({
model: "deepseek-chat",
messages: [{role: "user", content: prompt}]
})
});
return response.json();
}
// 后端代理示例(Node.js/Express)
app.post('/api/deepseek-proxy', async (req, res) => {
const response = await fetch('https://api.deepseek.com/v1/chat/completions', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${process.env.DEEPSEEK_API_KEY}`
},
body: JSON.stringify(req.body)
});
const data = await response.json();
res.json(data);
});
第二步 本地化部署须知
若需本地部署需满足:
- 企业级GPU服务器集群(至少8xA100 80G)
- 官方授权协议(DeepSeek尚未开源模型权重)
- 专业AI工程团队支持
第三步 完整技术栈示例(以Next.js为例):
// .env.local
DEEPSEEK_API_KEY=your_api_key
// pages/api/chat.js
export default async function handler(req, res) {
const { messages } = req.body;
const response = await fetch('https://api.deepseek.com/v1/chat/completions', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${process.env.DEEPSEEK_API_KEY}`
},
body: JSON.stringify({
model: "deepseek-chat",
messages,
temperature: 0.7
})
});
const data = await response.json();
res.status(200).json(data);
}
// 前端组件
import { useState } from 'react';
export default function ChatInterface() {
const [messages, setMessages] = useState([]);
const [input, setInput] = useState('');
const handleSubmit = async (e) => {
e.preventDefault();
const newMessages = [...messages, { role: 'user', content: input }];
setMessages(newMessages);
const response = await fetch('/api/chat', {
method: 'POST',
body: JSON.stringify({ messages: newMessages })
});
const { choices } = await response.json();
setMessages([...newMessages, choices[0].message]);
setInput('');
};
return (
<div className="chat-container">
{/* 聊天界面实现 */}
</div>
);
}